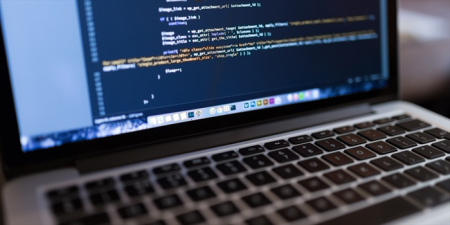
Why Use a PHP Framework?
PHP is one of the most widely used languages for web development. It is easy to program, has good performance, and offers great functionality to perform almost all the tasks required by a project.
Several PHP frameworks have emerged throughout the years and have established themselves as solid development aids for web applications. The most common pattern used by PHP frameworks is the Model View Controller (MVC). A PHP framework is a collection of tools and design patterns that offers ready-to-use PHP code to developers.
There are many advantages to using an MVC framework. The most common are outlined below.
Separation of Logic
With the MVC pattern, the PHP framework separates the application logic into three distinct areas:
- Model. All the database interactions
- View. All the HTML output or anything that needs to be sent to the browser
- Controller. All the business logic including the routing of requests, dispatching pages, etc.
Development Speed
An MVC framework supports a certain structure to allow a more rapid application development. The framework itself offers functionality right out of the box, which can be used with a few lines of code. Additionally, frameworks are often extendable, giving the developer the option of plugging in existing functionality as needed.
Smoother Learning Curve
An MVC framework is very well known in the community, making it easier for new developers coming into the project to get up to speed.
Stability & Standards
PHP is a very simple language. This is one of its great features, but also its biggest downfall, since inexperienced developers can write bad code without even realizing it. This issue becomes even more troubling whenever security is concerned. A framework like MVC forces developers to follow a certain coding pattern, which reduces the risk of errors. With clearly established rules, developers can collaborate better, share code, and work in different areas of the application without interfering with one another. Frameworks also make the adoption of Agile processes easier, since tasks and issues are streamlined and much easier to handle.
Single Entry Point
For MVC frameworks, there is one entry point for the application: one file. This file is responsible for bootstrapping the application, setting up configuration, database access, routing, and other necessary services. Because of that, making global changes to the application is much easier, and handling incoming data is easier and more secure.
Don’t Repeat Yourself (DRY)
Due to the structure and layout of the MVC framework, developers are able to carefully position code so that it can be reused multiple times in the application, thus removing duplication and reducing the risk of introducing unexpected behavior. Additionally, keeping the code DRY reduces maintenance time and in most cases makes the code execute more efficiently.
Form Validation
Another great time saver and solid programming technique is form validation in an application. Frameworks provide an easy interface to validate web form data ensuring that server validation is in place, adding one more layer to ensure that data is clean before moving deeper into the application.
Database Abstraction
MVC frameworks offer database abstraction. This allows the code to be structured and implemented in such a way that the application interfaces with the database using a common interface, while that interface is translated to the communication protocols that the database needs. By using this abstraction, developers can change the database with mere configuration changes and no additional application changes.
Input/Output Filtering
One of the core components of every MVC framework is the input/output filtering. MVC frameworks offer utility functions that sanitize input to ensure data is cleaned before being inserted in the database. Output can also be sanitized to ensure clean output.
Session and Cookie Handling
Another core component is the handling of session and cookies. PHP frameworks have easy-to-use functions that offer great flexibility in terms of accessing and storing session or cookie data.
Security
Security is one of the most important components in every PHP framework. Trying to implement security without the help of a framework is very difficult and often leads to omissions. Framework authors devote a lot of time to ensure that each application built within the PHP framework is as secure as possible. This is why, when security holes are discovered, framework authors immediately issue updates.
Template Engine
Separating the view layer from the application is an important step in decoupling an application. MVC frameworks ensure that the view component accepts data from the controllers (business logic) and presents it to the client (web browser). A template engine offers a more flexible approach in displaying data, without unnecessary repetition of code and with additional functionality such as loops for repeating data, conditionals, comparisons, etc.
Events Management
One of the most important components of the PHP framework is the Events Manager. The Events Manager is a collector of specific actions (events), which allows the framework to notify subscribed components (fire events). This pattern allows for a much simpler code and is very efficient, since only listeners registered to specific events will be called only as needed.
Access Control List
PHP frameworks also offer ACL implementations. As mentioned above, the application has one entry point and therefore can handle routing from one place. Each URL (or route) is considered to be one resource, which is allowed or denied based on the group of each user. This offers a much better implementation in terms of security and unauthorized access.
Unit Testing
The file and implementation structure of a PHP framework simplifies unit testing, too. The unit tests can be implemented to bootstrap the application and dispatch it within their run. Unit tests are essential to ensure a bug-free application (as much as possible), and certain frameworks have been developed with testing in mind.